Coding isn’t just about writing functional code; it’s also about how you write it. There are unspoken rules that guide developers, whether they are beginners or experts. These rules focus on clarity, collaboration, and maintaining best practices. Understanding them is key to becoming a more effective and respected developer.
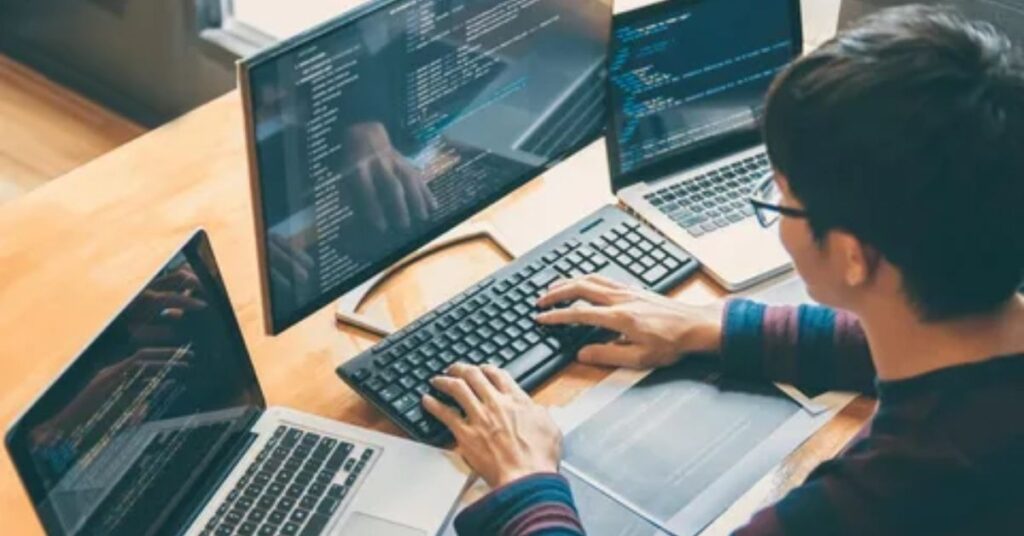
Coding has its unspoken rules that every developer, whether new or experienced, should know. These rules help improve efficiency and collaboration. Respect for others’ code, clean documentation, and consistent style matter. Following these guidelines makes coding smoother and more professional.
Also Red:http://SIA 588B AITimes: Exploring the Future of Technology
Understanding the Foundation of Coding Excellence
Coding excellence starts with mastering the basics. Understanding how to write clean, readable code is essential for any developer. This foundation helps in solving problems efficiently and makes collaboration easier.
Good practice involves following coding standards and keeping code simple. Avoiding unnecessary complexity helps maintain clarity and reduces errors. Consistency in naming conventions and structure also improves code readability.
Testing your code is another crucial aspect of coding excellence. It ensures that your code works as expected and prevents future issues. By continuously improving your skills and learning from mistakes, you can achieve coding excellence over time.
Write Code for Humans, Not Computers
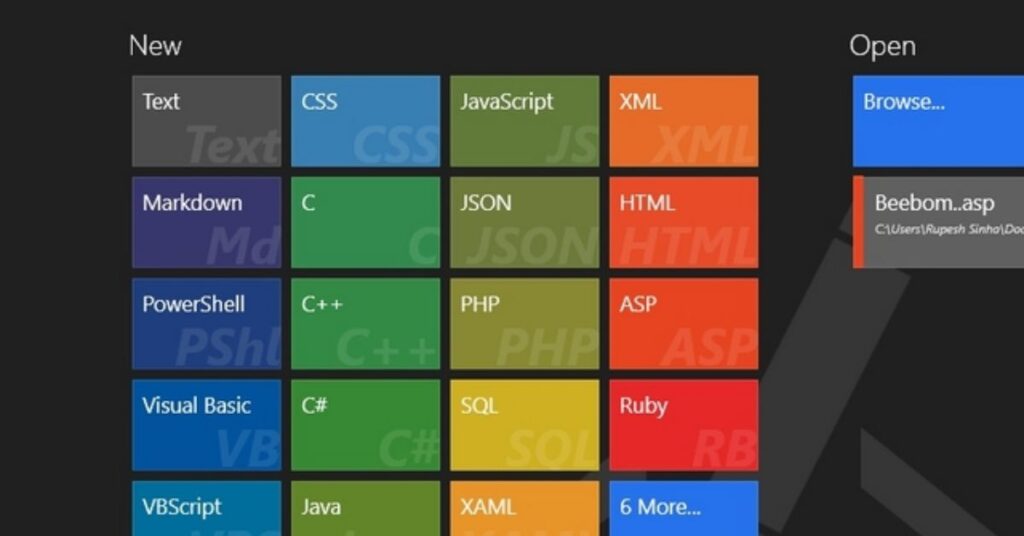
Writing code for humans focuses on clarity. When you write code, think about how easily someone else can read and understand it. Simple, clean code is always better than complicated, hard-to-follow solutions.
Using meaningful names for functions and variables helps others know exactly what they do. Comments should explain why something is done, not just how it works. This makes it easier for anyone reviewing your code to quickly understand your logic.
Collaboration is key in coding. Writing code that is understandable by others improves teamwork and reduces mistakes. Clear code saves time and makes debugging or updating easier for everyone involved.
Comments Should Explain Why, Not What
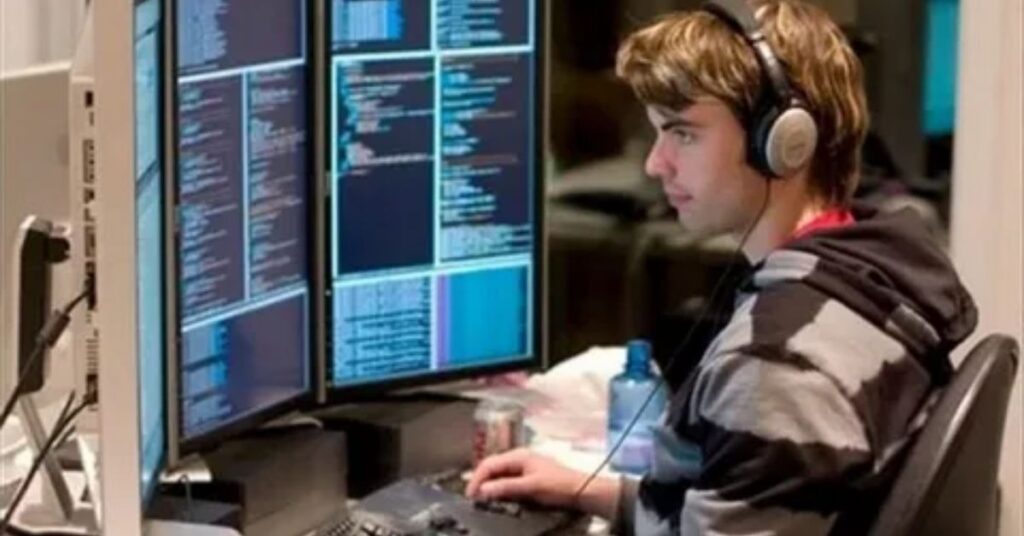
Comments in code should focus on explaining why something is done, not what the code does. The “what” is usually clear from well-written code itself. For example, instead of saying, “This adds two numbers,” explain, “Adding these numbers ensures accurate tax calculation.” This helps others understand the purpose behind decisions. Good comments save time and reduce confusion for future developers.
Understand the Code’s Purpose: Before writing a comment, know why the code exists and what problem it solves.
Avoid Describing the Obvious: Don’t explain what the code does if it’s already clear from the code itself.
Focus on Intent: Write comments that explain the reason behind the logic or decision.
Be Clear and Concise: Use simple language to communicate the “why” without overcomplicating.
Update Comments as Code Changes: Ensure comments always reflect the current logic and purpose of the code.
Step | Description |
Understand the Purpose | Know the problem the code solves and the reason for its implementation. |
Avoid Obvious Details | Don’t restate what the code already clearly shows; focus on adding value. |
Explain Intent | Provide context for the logic or decisions, such as trade-offs or design choices. |
Be Clear and Concise | Use simple, precise language to explain the “why” without overloading with details. |
Keep Comments Updated | Regularly review and edit comments to ensure they align with the current state of the code. |
Don’t Repeat Yourself (DRY)
The DRY principle, meaning “Don’t Repeat Yourself,” is a rule that encourages avoiding duplicate code. Instead of copying and pasting, write reusable parts like functions, modules, or classes. This makes your code easier to manage and reduces errors.
When code is repeated, any change has to be made in multiple places. This increases the chances of missing something, leading to bugs and inconsistencies. DRY helps prevent these issues by centralizing logic in one place.
Reusable code saves time during development and makes updates faster. If a feature needs improvement, you only update one section, and the changes apply everywhere. This keeps the project more organized.
Following DRY also improves teamwork. Other developers can quickly understand your structure, making collaboration smoother. Clean, efficient, and consistent code benefits everyone working on the project.
Learn to Fail Gracefully (Error Handling)
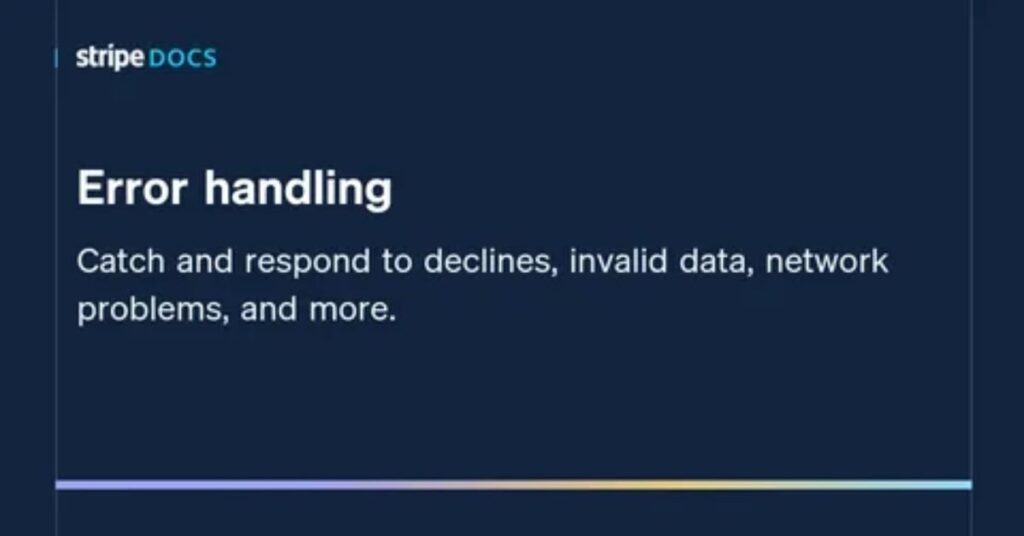
Learning to fail gracefully means handling errors in a way that doesn’t crash your program. Instead of stopping completely, the code should respond with a helpful message or alternative action. This improves the user’s experience.
Good error handling starts with anticipating problems. Use checks to catch potential issues, like invalid inputs or missing files, before they cause bigger failures. Always test your code for edge cases.
When an error occurs, provide clear messages to explain what went wrong. Avoid confusing technical terms; use simple language that helps users or developers fix the issue quickly.
Failing gracefully also means logging errors for future debugging. This allows developers to find and fix problems more easily, ensuring the system remains reliable and efficient
Test Early and Often
Testing early and often is a crucial practice in development. By testing your code after writing small sections, you can catch mistakes quickly and avoid bigger issues later. It helps identify problems before they become complex or harder to fix.
When you test frequently, you ensure that each part works as expected and doesn’t break other areas of your code. This approach also builds confidence that the final product will be stable. Regular testing saves time in the long run and makes the development process smoother.
Refactor Regularly
Refactoring means improving your code without changing its behavior. Regularly cleaning up your code makes it easier to understand and maintain.
Over time, as you add new features, your code can become messy. Refactoring helps remove unnecessary parts and simplifies complex sections, making the code more efficient.
By refactoring often, you ensure that your project remains clean and flexible. It reduces technical debt and makes future changes or debugging easier. A well-organized codebase improves both development speed and code quality.
Use Version Control Properly
Version control helps track changes in your code over time. Using tools like Git allows you to save versions, so you can easily go back if something goes wrong.
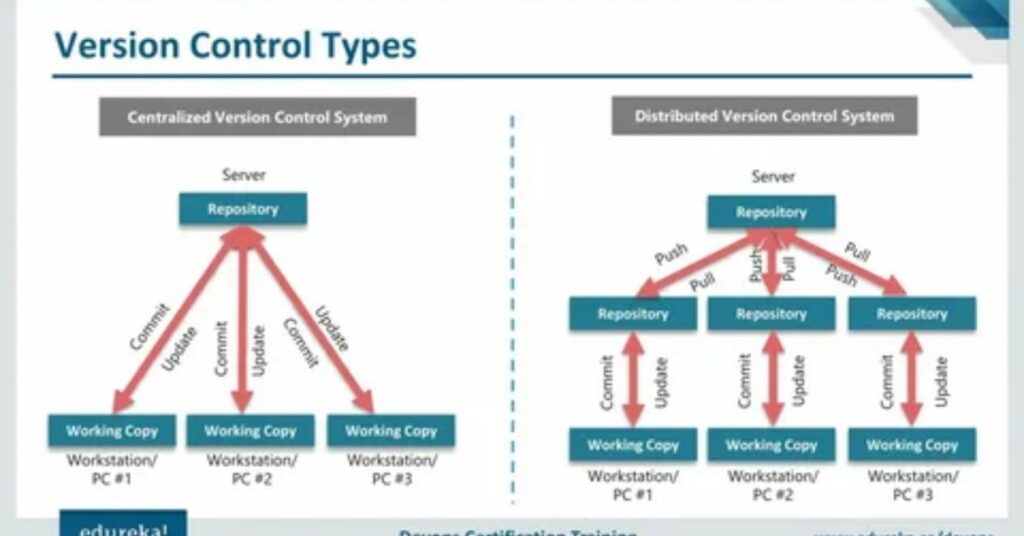
By using version control properly, you collaborate better with teammates and avoid overwriting each other’s work. It helps organize your workflow and keeps the project history clear, making it easier to spot and fix errors.
Choose a Version Control System: Start by selecting a tool like Git to track changes in your code.
Commit Often: Save your work regularly with clear messages that explain what changes you made.
Use Branches: Create separate branches for different features or fixes to keep the main code clean.
Merge Carefully: When merging changes, review the code to avoid conflicts or mistakes.
Collaborate and Sync: Share your changes with others by pushing to a shared repository, and always pull the latest updates.
Know When to Stop Optimizing
Knowing when to stop optimizing is key to balancing efficiency and development time. It’s easy to keep improving code, but sometimes further optimization offers little benefit and wastes time.
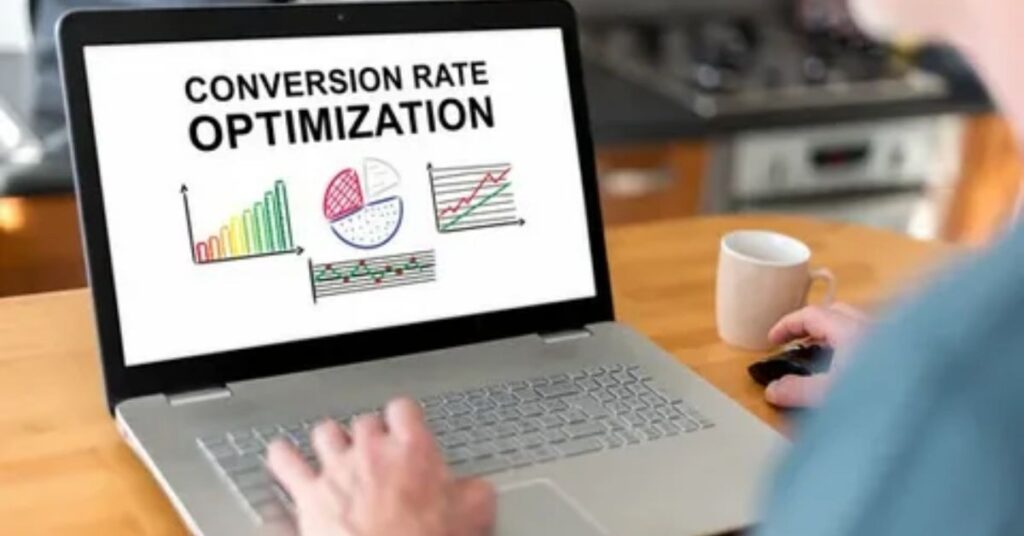
Focus on optimizing the parts that matter most, like performance bottlenecks. Once the code meets the required standards, stop optimizing and move on to other tasks to keep the project on track
Step | Description |
dentify Key Areas | Focus on optimizing the parts of code that have the biggest impact on performance. |
Set Clear Goals | Define what success looks like for optimization to avoid unnecessary changes. |
Measure Impact | Test before and after performance to ensure the optimization provides real improvements. |
Balance Time and Benefit | Consider the time spent on optimization versus the benefits gained, and avoid diminishing returns. |
Know When to Move On | Once you’ve optimized effectively, stop and shift focus to other important tasks in the project. |
Understand Your Tools and Ecosystem
Understanding your tools and ecosystem is crucial for effective development. Knowing how the tools you use work allows you to make better decisions and solve problems faster.
It’s important to stay familiar with the libraries, frameworks, and environments you’re working with. This helps you take full advantage of their features and avoid common pitfalls.
By mastering your tools, you can improve efficiency, write cleaner code, and reduce errors. Being comfortable with your ecosystem also helps you troubleshoot and adapt to changes more easily.
Communicate Effectively with Your Team
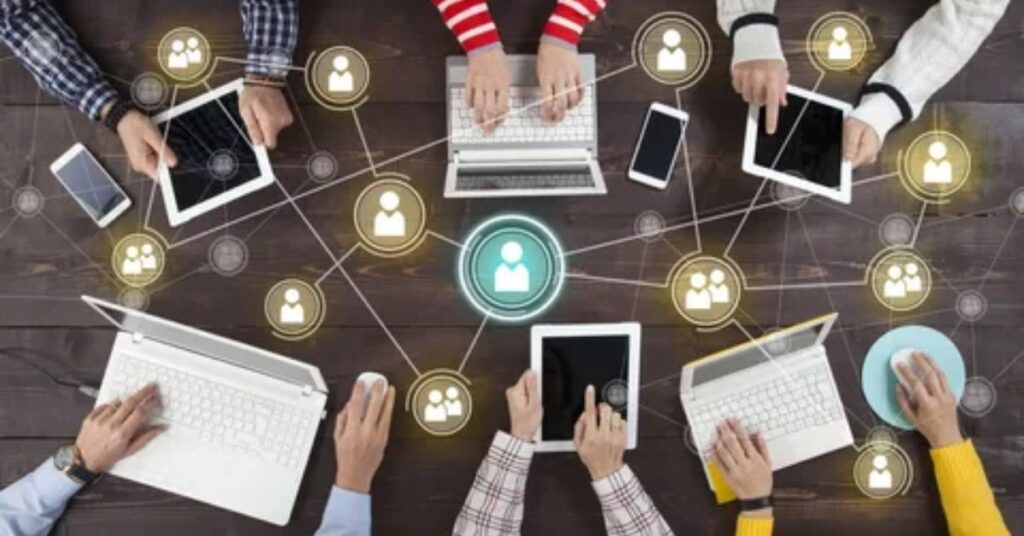
Effective communication with your team helps everyone stay on the same page. It’s important to share ideas clearly and listen to others’ input.
Use simple language and avoid jargon to make your messages easy to understand. Regular check-ins and updates ensure everyone knows their tasks and deadlines.
Good communication builds trust and improves collaboration. When team members feel heard and informed, the project runs more smoothly and is more likely to succeed.
Don’t Fear Technical Debt—Manage It
Technical debt refers to shortcuts or quick fixes in your code that may cause problems later. While it’s important to avoid accumulating too much, some debt is inevitable in fast-paced projects.
The key is to manage it wisely. Regularly refactor your code and address technical debt when it becomes a problem, rather than ignoring it.
By keeping track of technical debt, you can prevent it from piling up and slowing down your development. When handled well, it won’t negatively impact the project’s progress or quality.
The Path to Continuous Growth
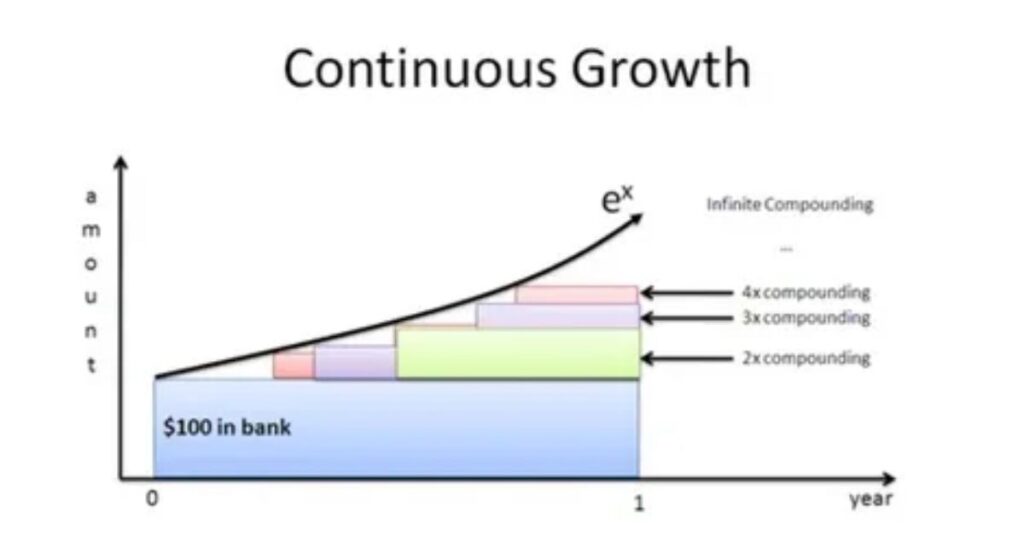
The path to continuous growth in coding involves always learning and improving. Stay curious, explore new technologies, and practice regularly to keep your skills sharp.
By seeking feedback and embracing challenges, you can overcome obstacles and develop better problem-solving abilities. Continuous growth makes you more adaptable and prepares you for future opportunities in tech
Ask for Help and Collaborate
Asking for help is a key part of learning and growing as a developer. Don’t hesitate to reach out when you’re stuck or unsure about something.
Collaboration with others can bring fresh ideas and solutions. Working together not only speeds up the process but also improves the quality of the final product.
Keep Your Codebase Organized
Keeping your codebase organized makes it easie to navigate and maintain. Use clear naming conventions and structure your files logically to avoid confusion.
Regularly refactor your code to remove unnecessary parts and improve clarity. This keeps your project neat and helps new developers understand it faster.
An organized codebase also helps you spot issues quickly and work more efficiently. When everything is in its right place, it reduces errors and makes future updates simpler.
Stay Up-to-Date, But Don’t Chase Every Trend
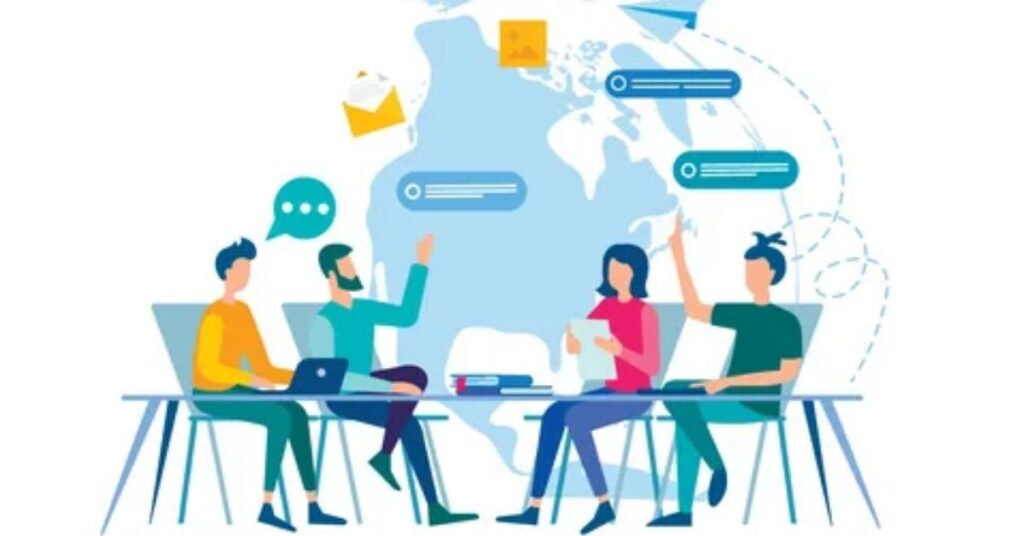
Staying up-to-date with new technologies is important, but it’s not necessary to follow every trend. Focus on tools and techniques that suit your project and needs.
Chasing every new trend can waste time and distract from solving real problems. Choose to learn things that add value and align with your long-term goals in development.
Also Red:http://SIA 588B AITimes: Exploring the Future of Technology
Frequently Asked Questions
Refining Technical Expertise
Intermediate developers deepen their knowledge in specific areas, enhancing their ability to solve complex problems. This growth helps them handle more challenging tasks with greater ease.
Developing Professional Testing Skills:
At this stage, developers learn to write efficient tests, detect bugs early, and ensure high-quality code. Testing skills improve their problem-solving and debugging capabilities.
Becoming Effective Team Players:
Intermediate developers improve communication and collaboration within teams. They understand the importance of sharing ideas and actively contributing to group discussions.
Learning to Manage Time:
Intermediate developers focus on balancing multiple tasks and meeting deadlines. They begin to prioritize efficiently and work under pressure while maintaining quality.
Building Leadership Abilities
Developers at this level start mentoring juniors, guiding them with technical advice. This helps build leadership skills and prepares them for future roles in management or architecture
Summery
The unspoken rules of coding help developers at all levels write better code. Beginners focus on learning clean and efficient coding practices. Experienced developers refine their skills by improving collaboration and testing. Clear communication and problem-solving are key for everyone. Following these rules leads to better code and continuous growth as a developer.